Advanced example#
This jupyter notebook is a complete example of how to use Watlab. It will guide you through the different steps of a classic Watlab usage: the mesh creation with GMSH, the model description with one of the modules of Watlab, and the visualisation with the visualisation tools of Watlab.
Now make sure you have installed Watlab correctly, and dig in with us!
All you need is GMSH!#
GMSH is a mesh generator. It comes with a GUI, but also with a nice Python-API. The very first step is to import GMSH and initialise the process. Then, we’ll be able to construct the mesh point by point.
[1]:
import gmsh
import sys
gmsh.initialize()
gmsh.model.add("advancedDamBreak")
Then, you need to add points and lines to the gmsh model in order to generate the domain:
[2]:
lc = 0.01
gmsh.model.geo.addPoint(0 , 0 , 0, lc, 1)
gmsh.model.geo.addPoint(0.5, 0 , 0, lc, 2)
gmsh.model.geo.addPoint(1 , 0 , 0, lc, 3)
gmsh.model.geo.addPoint(0 , 0.4, 0, lc, 4)
gmsh.model.geo.addPoint(0.5, 0.4, 0, lc, 5 )
gmsh.model.geo.addPoint(1 , 0.4, 0, lc, 6 )
gmsh.model.geo.addPoint(0.5, 0.6, 0, lc, 7 )
gmsh.model.geo.addPoint(1 , 0.6, 0, lc, 8 )
gmsh.model.geo.addPoint(0.7, 0.1, 0, lc, 9 )
gmsh.model.geo.addPoint(0.8, 0.1, 0, lc, 10)
gmsh.model.geo.addPoint(0.7, 0.3, 0, lc, 11)
gmsh.model.geo.addPoint(0.8, 0.3, 0, lc, 12)
gmsh.model.geo.addLine(1, 2, 1)
gmsh.model.geo.addLine(2, 3, 2)
gmsh.model.geo.addLine(1, 4, 3)
gmsh.model.geo.addLine(2, 5, 4)
gmsh.model.geo.addLine(3, 6, 5)
gmsh.model.geo.addLine(4, 5, 6)
gmsh.model.geo.addLine(5, 7, 7)
gmsh.model.geo.addLine(7, 8, 8)
gmsh.model.geo.addLine(8, 6, 9)
gmsh.model.geo.addLine(10, 9, 10)
gmsh.model.geo.addLine(9, 11, 11)
gmsh.model.geo.addLine(11, 12, 12)
gmsh.model.geo.addLine(12, 10, 13)
[2]:
13
From the different lines, you can build curved loops and planar surfaces:
[3]:
gmsh.model.geo.addCurveLoop([1, 4, -6, -3], 1)
gmsh.model.geo.addCurveLoop([2, 5, -9, -8, -7, -4], 2)
gmsh.model.geo.addCurveLoop([10, 11, 12, 13], 3)
gmsh.model.geo.addPlaneSurface([1], 1)
gmsh.model.geo.addPlaneSurface([2,3], 2)
[3]:
2
The physical entities are used to describe boundaries and surfaces:
[4]:
gmsh.model.geo.synchronize()
gmsh.model.addPhysicalGroup(1, [1,2],name="Boundaries down")
gmsh.model.addPhysicalGroup(1, [5,9],name="Boundaries right")
gmsh.model.addPhysicalGroup(1, [6,8],name="Boundaries up")
gmsh.model.addPhysicalGroup(1, [3,7],name="Boundaries left")
gmsh.model.addPhysicalGroup(1, [10,11,12,13],name="Boundaries box")
gmsh.model.addPhysicalGroup(2, [1],name="Filled area")
gmsh.model.addPhysicalGroup(2, [2],name="Empty area")
[4]:
7
Now, you only need to generate your mesh:
[5]:
gmsh.model.mesh.generate(2)
gmsh.model.mesh.optimize('Laplace2D')
gmsh.write("advancedDamBreakMesh.msh")
if '-nopopup' not in sys.argv:
gmsh.fltk.run()
gmsh.finalize()
Watlab helps you master the model#
Now that the geometry of your domain is done, you can focus on solving your hydraulic problem. For this, you need a solver (hopefully, Watlab provides you one!). You also need to tell Watlab what mesh represents the domain, and what conditions are set to the cells and the boundaries.
The example here is only hydrodynamics, so the solver is hydroflow.
In this example, we use a the HydroFlow’s API to model another geometrical case. It consists of a dambreak against a more complex geometry with a 90° angle and an obstacle
First, import Watlab, import the mesh, and create a Watlab model!
[6]:
import watlab
mesh = watlab.Mesh("advancedDamBreakMesh.msh")
model = watlab.HydroflowModel(mesh)
Several properties can be given to the model (see the documentation for a complete set of properties).
[7]:
model.name = "Advanced Dam Break"
model.ending_time = 5
model.Cfl_number = 0.95
Initial conditions and physical properties, such as friction coefficient or water level can be given as follows:
[8]:
model.set_friction_coefficient("Filled area",0.0)
model.set_friction_coefficient("Empty area",0.0)
model.set_initial_water_level("Filled area", 0.15)
model.set_initial_water_level("Empty area", 0.015)
model.set_wall_boundaries(["Boundaries down","Boundaries up","Boundaries left","Boundaries right","Boundaries box"])
Provide the number of required results files:
[9]:
model.set_picture_times(n_pic = 51)
Finally, we can specify output parameters, generate the data files and run this specific model:
[10]:
model.export_data()
print("Input files generated!")
model.solve()
Input files generated!
hydroflow.exe exists
Launching the executable ...
Total execution time: 49 sec. for 5 sec. of simulation.
Visualize the results with the Plotter class combined with matplotlib#
[12]:
import matplotlib.pyplot as plt
#create the mesh and plotter object
plotter = watlab.Plotter(mesh)
#time of the plot
time0 = "0_00"
time1 = "1_20"
time2 = "2_90"
time3 = "3_60"
#path of the pic at the corresponding times
myPic0 = "output\\pic_"+ time0 +".txt"
myPic1 = "output\\pic_"+ time1 +".txt"
myPic2 = "output\\pic_"+ time2 +".txt"
myPic3 = "output\\pic_"+ time3 +".txt"
#plot
plotter.plot(myPic0, "h",colorbar_values=[0,0.15])
plt.title("Time: " + time0 +" s")
plt.show()
plotter.plot(myPic1, "h")
plt.title("Time: " + time1 +" s")
plt.show()
plotter.plot(myPic2, "h")
plt.title("Time: " + time2 +" s")
plt.show()
plotter.plot(myPic3, "h")
plotter.show_velocities(myPic3, scale=100)
plt.title("Time: " + time3 +" s")
plt.show()
plotter.plot(myPic3, "h")
plotter.show_velocities(myPic3, scale=7)
plt.title("Zoom at time: " + time3+" s")
plt.xlim([0.7, 0.9])
plt.ylim([0,0.2])
plt.show()
#cross section plot
plotter.plot_profile_along_line(myPic0, "h", x_coordinate=[0, 1], y_coordinate=[0.2,0.2], new_fig=True, label=time0)
plt.show()
plotter.plot_profile_along_line(myPic1, "h", x_coordinate=[0, 1], y_coordinate=[0.2,0.2], label=time1)
plt.show()
plotter.plot_profile_along_line(myPic2, "h", x_coordinate=[0, 1], y_coordinate=[0.2,0.2], label=time2)
plt.show()
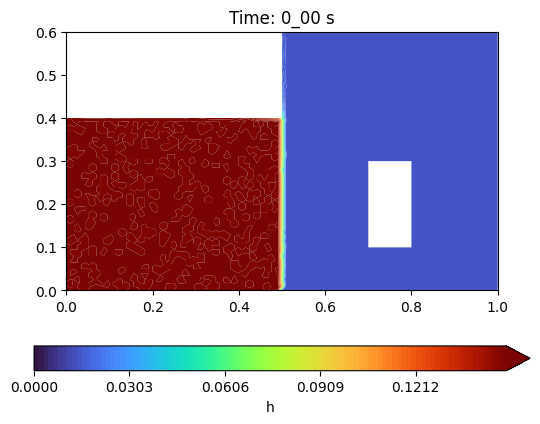
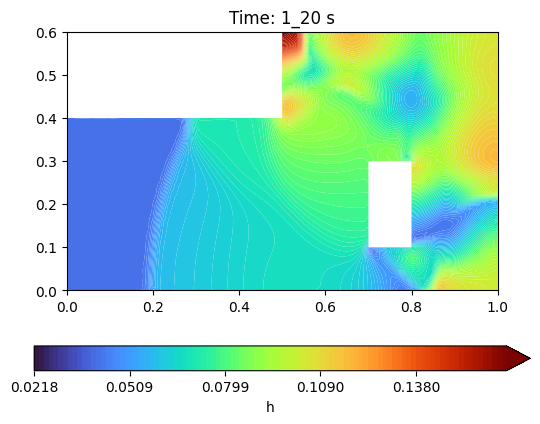
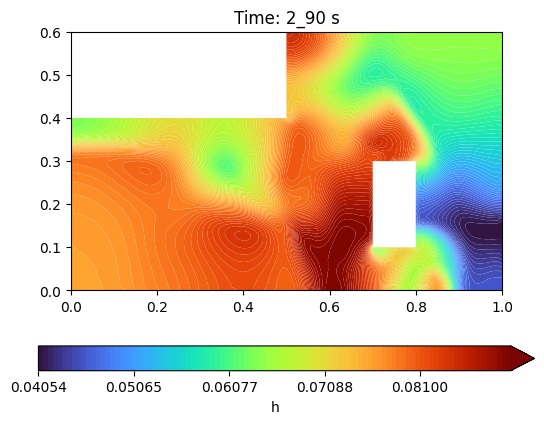
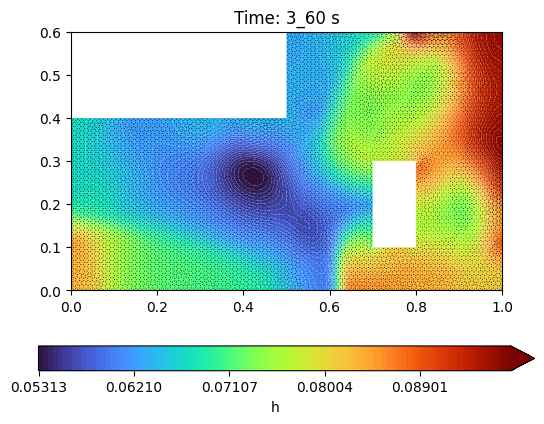
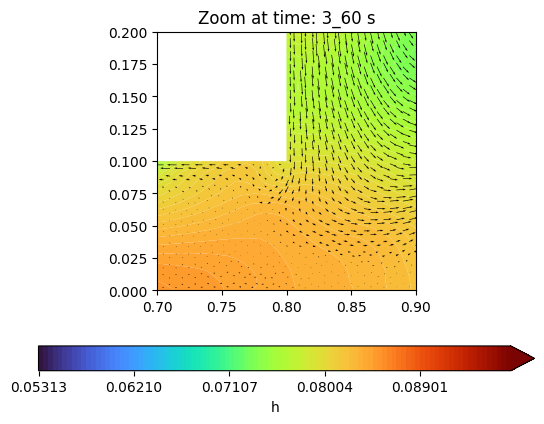
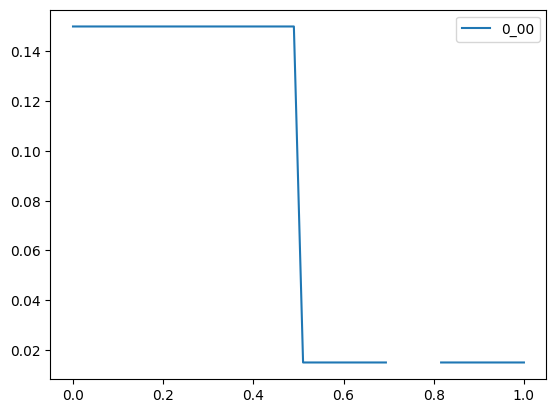
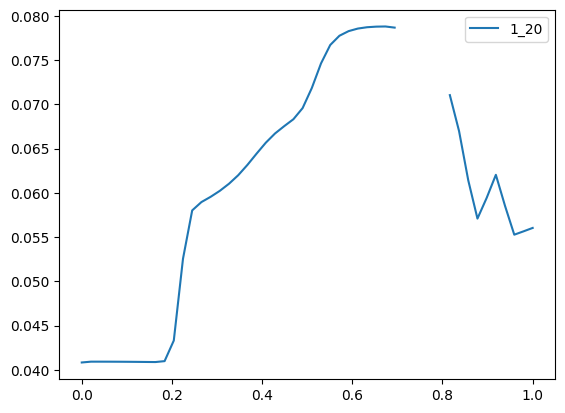
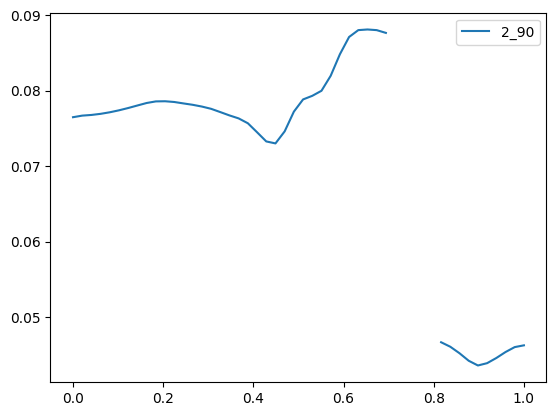
[ ]: